One of the nice features in HTML5 is the abililty to specify a specific input type for HTML text input boxes. There a host of very useful input types available including email, number, date, datetime, month, number, range, search, tel, time, url and week. For a more complete list you can check out the MDN reference. Date input types also support automatic validation which can be useful in some scenarios but maybe can get in the way at other times.
One of the more common input types, and one that can most benefit of a custom UI for selection is of course date input. Almost every application could use a decent date representation and HTML5's date input type seems to push into the right direction. It'd be nice if you could just say:
<form action="DateTest.html">
<label for="FromDate">Enter a Date:</label>
<input type="date" id="FromDate" name="FromDate"
value="11/08/2012" class="date" /> <hr />
<input type="submit" id="btnSubmit" name="btnSubmit"
value="Save Date" class="smallbutton" />
</form>
but if you'd expect to just work, you're likely to be pretty disappointed.
Problem #1: Browser Support
For starters there's browser support. Out of the major browsers only the latest versions of WebKit and Opera based browsers seem to support date input. Neither FireFox, nor any version of Internet Explorer (including the new touch enabled IE10 in Windows RT) support input type=date. Browser support is an issue, but it would be OK if it wasn't for problem #2.
Problem #2: Date Formatting
If you look at my date input from before:
<input type="date" id="FromDate" name="FromDate"
value="11/08/2012" class="date" />
You can see that my date is formatted in local date format (ie. en-us). Now when I run this sadly the form that comes up in Chrome (and also iOS mobile browsers) comes up like this:
Chrome isn't recognizing my local date string. Instead it's expecting my date format to be provided in ISO 8601 format which is:
2012-11-08
So if I change the date input field to:
<input type="date" id="FromDate" name="FromDate"
value="2012-10-08" class="date" />
I correctly get the date field filled in:
Also when I pick a date with the DatePicker the date value is also returned is also set to the ISO date format. Yet notice how the date is still formatted to the local date time format (ie. en-US format). So if I pick a new date:
and then save, the value field is set back to:
2012-11-15
using the ISO format. The same is true for Opera and iOS browsers and I suspect any other WebKit style browser and their date pickers.
So to summarize input type=date:
- Expects ISO 8601 format dates to display intial values
- Sets selected date values to ISO 8601
Now what?
This would sort of make sense, if all browsers supported input type=date. It'd be easy because you could just format dates appropriately when you set the date value into the control by applying the appropriate culture formatting (ie. .ToString("yyyy-MM-dd") ). .NET is actually smart enough to pick up the date on the other end for modelbinding when ISO 8601 is used. For other environments this might be a bit more tricky.
input type=date is clearly the way to go forward. Date controls implemented in HTML are going the way of the dodo, given the intricacies of mobile platforms and scaling for both desktop and mobile. I've been using jQuery UI Datepicker for ages but once going to mobile, that's no longer an option as the control doesn't scale down well for mobile apps (at least not without major re-styling). It also makes a lot of sense for the browser to provide this functionality - creating a consistent date input experience across apps only makes sense, which is why I find it baffling that neither FireFox nor IE 10 deign it necessary to support date input natively.
The problem is that a large number of even the latest and greatest browsers don't support this. So now you're stuck with not knowing what date format you have to serve since neither the local format, nor the ISO format works in all cases.
Modernizr
It's possible to check for the availability of input types with Modernizr by checking:
Modernizr.inputtypes.date
for true or false. Unfortunately this is a client side thing, which means you'd have to delay formatting your date on the client using some sort of client library for date formatting like Moment.js rather than pushing a date into the UI from the server. This would work for a client heavy application that's using client side templates to render content, but not so well for a typical HTML server rendered app.
Compromise?
For my current app I just broke down and used the ISO format and so I'll live with the non-local date format.
<input type="date" id="ToDate" name="ToDate"
value="2012-11-08" class="date"/>
Here's what this looks like on Chrome:
Here's what it looks like on my iPhone:
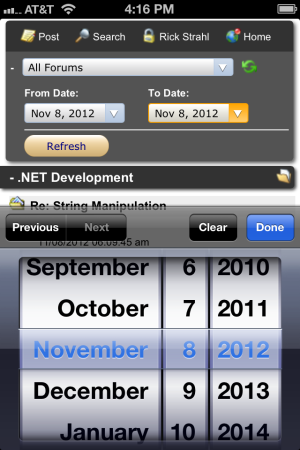
Both Chrome and the phone do this the way it should be. The iPhone date display and date picker in particular are very nice (better than anything on the desktop - that's for sure) and it demonstrates why we'd want this implemented in the browser to share this common UI for any application that provides date input. The iOS built-in date picker there certainly beats manually trying to edit the date using finger gymnastics.
Finally here's what the date looks like in FireFox which doesn't support date input types:
Certainly this is not the ideal date format, but it's clear enough I suppose. If users enter a date in local US format and that works as well (but won't work for other locales). It'll have to do.
Over time one can only hope that other browsers will finally decide to implement this functionality natively to provide a unique experience. Until then, incomplete solutions it is.
Related Posts
Other Posts you might also like