Here’s a quick and dirty tip if you’re dealing with JSON strings that you at times need to display for debugging or simply seeing a quick view of data represented. It may be that you have an application that captures HTTP responses and you need to actually decipher the JSON that was sent to you, or you’re creating a quick and dirty admin form where you just want to dump some settings information into a screen.
As is usually the case, JSON.NET makes JSON manipulations super easy – in fact it’s a single line of code:
string jsonFormatted = JValue.Parse(json).ToString(Formatting.Indented);
Here’s how you can test this out:
[TestMethod]
public void PrettifyJsonStringTest()
{
var test = new
{
name = "rick",
company = "Westwind",
entered = DateTime.UtcNow
};
string json = JsonConvert.SerializeObject(test);
Console.WriteLine(json); // single line JSON string
string jsonFormatted = JValue.Parse(json).ToString(Formatting.Indented);
Console.WriteLine(jsonFormatted);
}
The code above of course is contrived as SerializeObject() also supports the Formatting.Indented option. But assume for a second that you are getting data already in string format from somewhere such as an HTTP stream or a file on disk. You then use the above code to convert into something much more readable.
In practice, it’s nice if you have any interfaces that need to display JSON in the UI. For example in West Wind Web Surge I display sent and captured HTTP content and if the result is JSON the default Raw Response output looks like this:
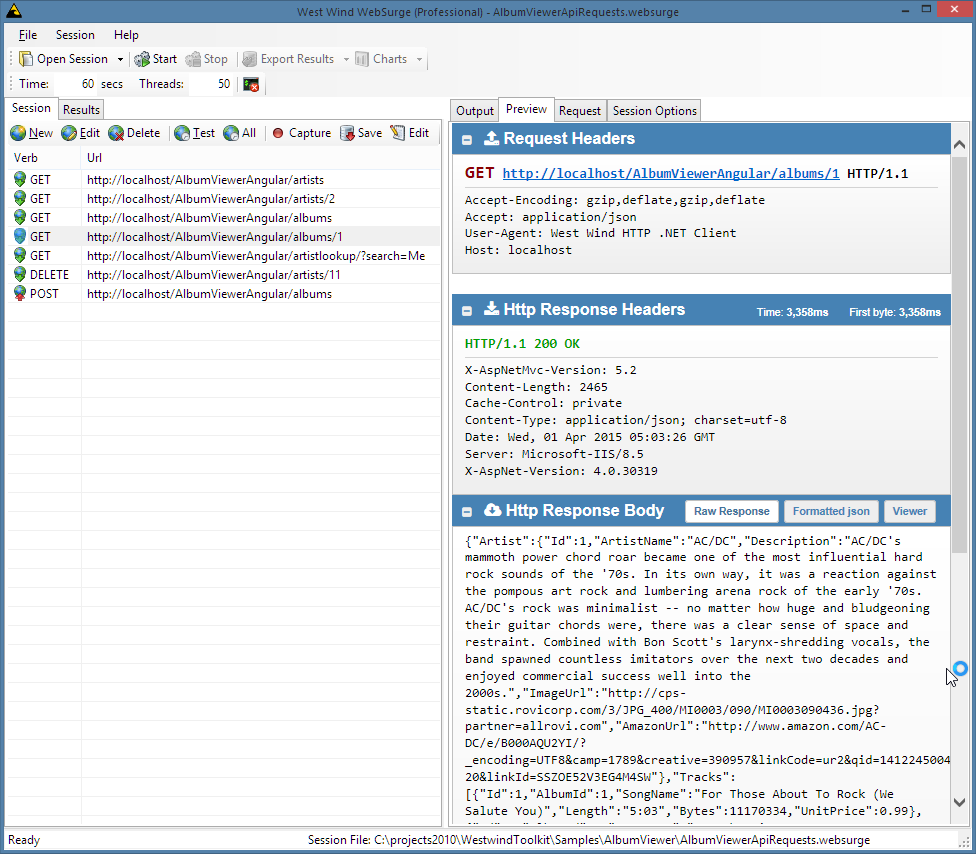
Workable but not exactly readable.
By applying formatting it sure is a lot easier to see what the JSON actually looks like:
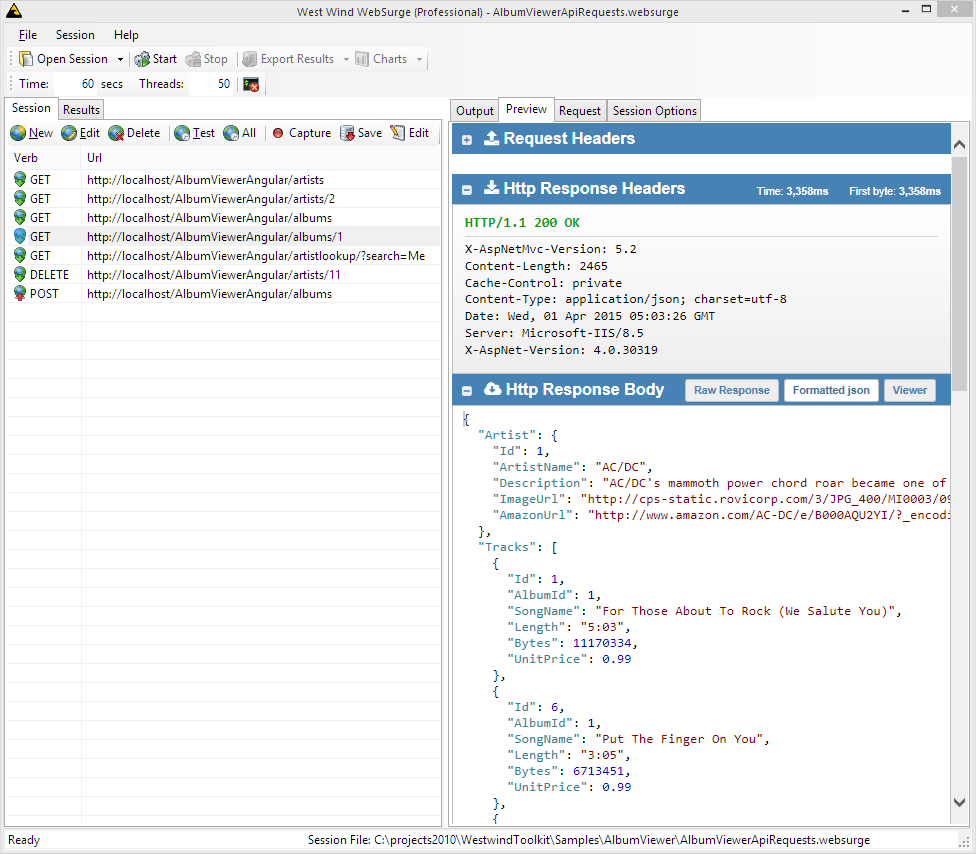
Likewise if you’re dealing with objects that have .ToString()
methods that return JSON (as many online SDKs do!), it’s nice to have an easy way to format the result into something more readable that you can dump into the Debug console.
It’s simple solution to a not so often required problem, but I’ve run into this enough times when I forgot exactly which JSON.NET object is required to handle this, so I wrote it down for next time for me to remember and maybe this might be useful to some of you as well.
Other Posts you might also like